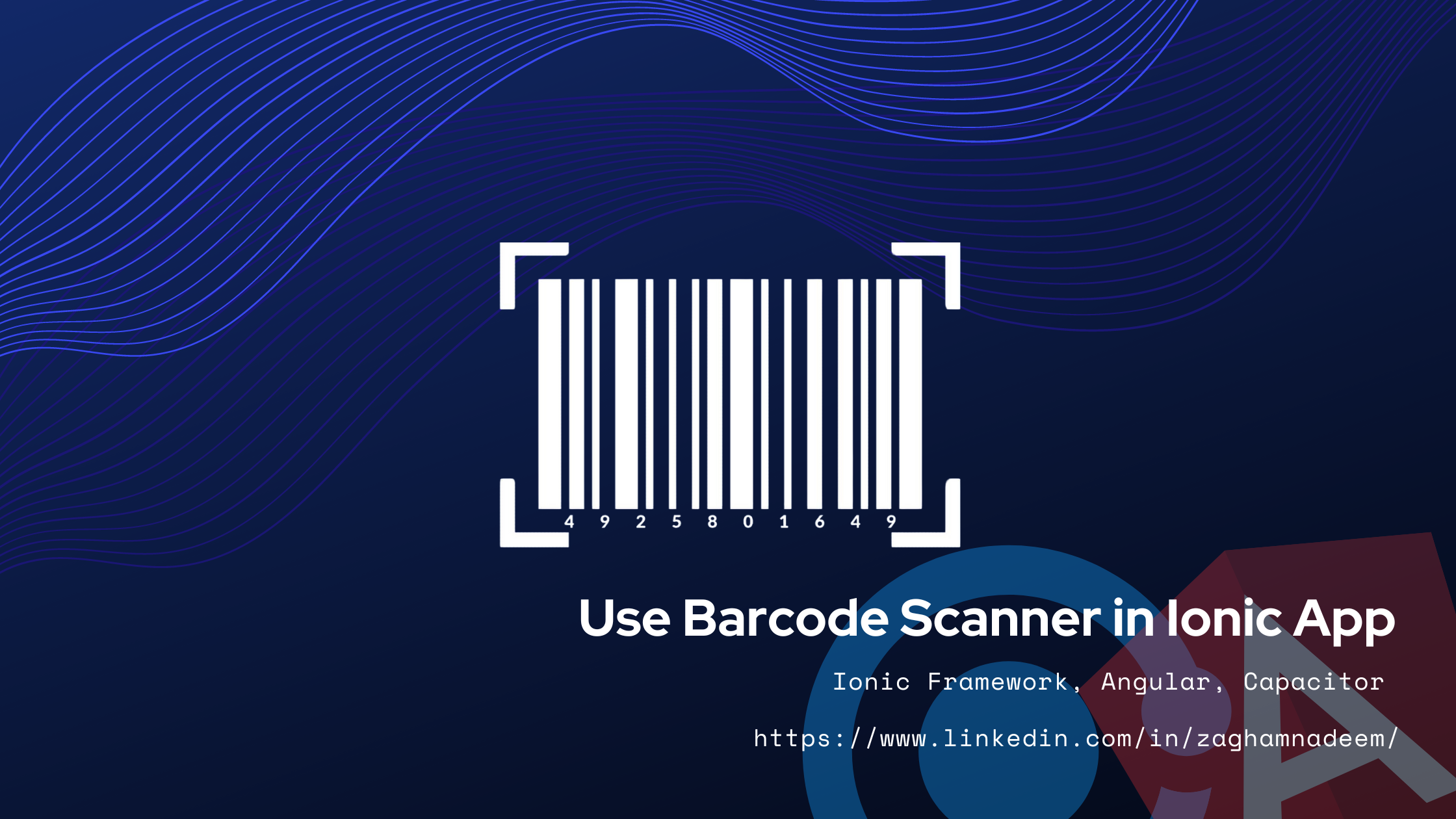
Barcode Scanner in Your Ionic App Using Capacitor
- Published on
- 8 mins
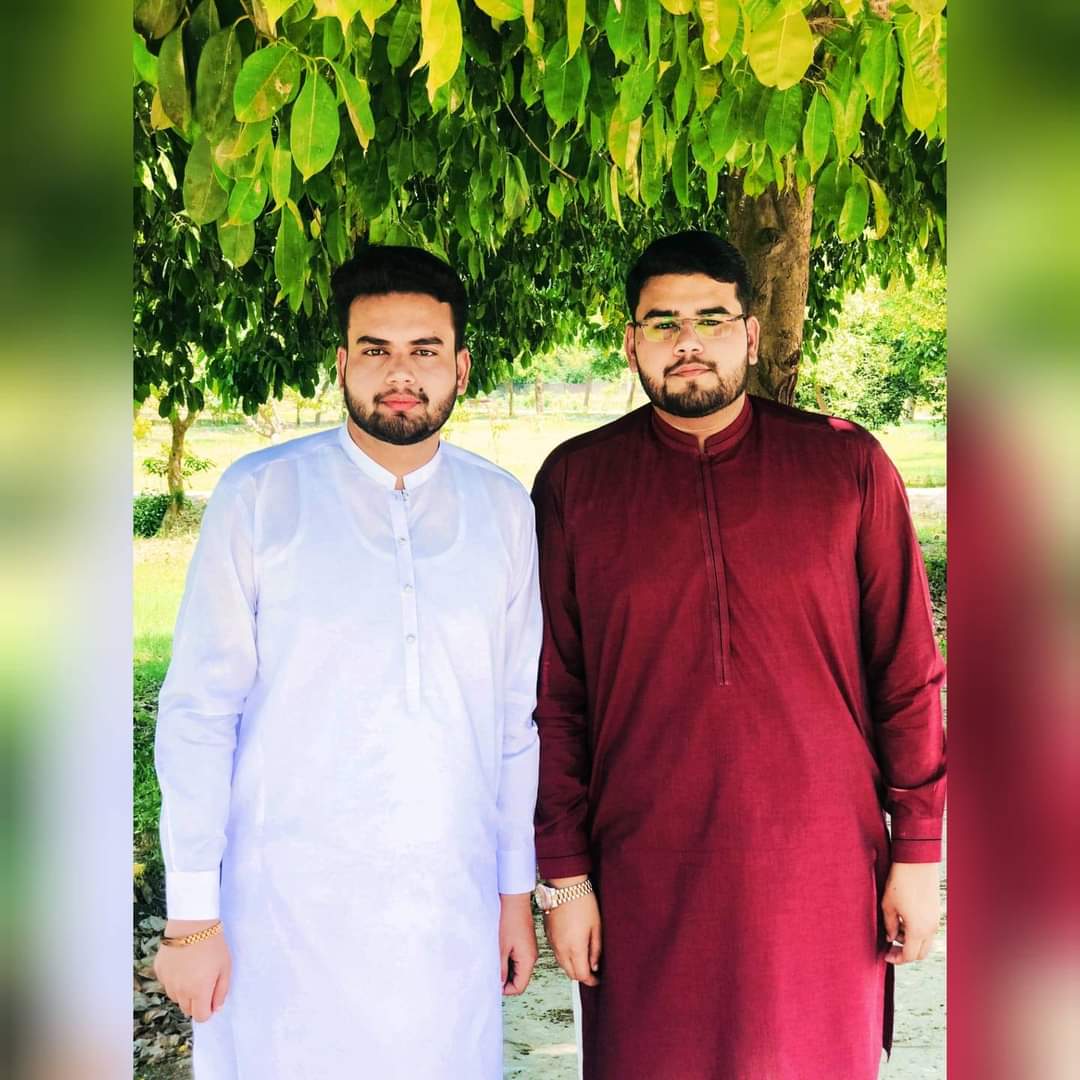
CEO & Founder
Integrating barcode scanning into your mobile app can significantly enhance functionality, making data entry faster and reducing errors. This guide will walk you through setting up barcode scanning in your Ionic app using the Capacitor plugin @capacitor/barcode-scanner
, which leverages Outsystems Barcode libraries.
Prerequisites
Before we dive into the setup, ensure you have the following prerequisites:
- Node.js and npm installed on your machine.
- Ionic CLI installed globally (
npm install -g @ionic/cli
). - Basic knowledge of Ionic and Angular.
Step 1: Create a New Ionic Project
Start by creating a new Ionic project. Open your terminal and run the following command:
ionic start barcode-scanner-app blank --type=angular --capacitor --package-id=com.zagham.barcode
You will be asked:
Would you like to build your app with NgModules or Standalone Components?
For this guide, we will use Standalone Components. However, you can choose NgModules if you prefer.
Navigate into your project directory:
cd barcode-scanner-app
Step 2: Install the Barcode Scanner Plugin
Install the barcode scanner plugin using npm:
npm install @capacitor/barcode-scanner
npx cap sync
Step 3: Implement Barcode Scanning Functionality
Open src/app/home/home.page.ts
and implement the barcode scanning functionality. First, import and inject the BarcodeScanner:
import { Component } from '@angular/core';
import { IonHeader, IonToolbar, IonTitle, IonContent, IonButton, IonText } from '@ionic/angular/standalone';
import { CapacitorBarcodeScanner, CapacitorBarcodeScannerOptions, CapacitorBarcodeScannerTypeHintALLOption } from '@capacitor/barcode-scanner';
@Component({
selector: 'app-home',
templateUrl: 'home.page.html',
styleUrls: ['home.page.scss'],
standalone: true,
imports: [IonButton, IonHeader, IonToolbar, IonTitle, IonContent, IonText],
})
export class HomePage {
public barcodeResult!: string;
private options: CapacitorBarcodeScannerOptions = {
scanButton: true,
hint: CapacitorBarcodeScannerTypeHintALLOption.ALL
};
public constructor() {}
public async scanBarcode(): Promise<void> {
this.barcodeResult = (await CapacitorBarcodeScanner.scanBarcode(this.options)).ScanResult;
console.log('Barcode data:', this.barcodeResult);
}
}
Step 4: Add a Button to Trigger Scanning
Open src/app/home/home.page.html
and add a button to trigger the barcode scanner:
<ion-header [translucent]="true">
<ion-toolbar>
<ion-title>Barcode Scanner</ion-title>
</ion-toolbar>
</ion-header>
<ion-content [fullscreen]="true">
<ion-text>
<h2>Barcode data: {{ barcodeResult ? barcodeResult : 'No data found' }}</h2>
</ion-text>
<ion-button expand="block" (click)="scanBarcode()">Scan Barcode</ion-button>
</ion-content>
Step 5: Add Android Platform
npm install @capacitor/android
npx cap add android
Step 6: Configure Android Platform
The barcode scanner plugin requires a minimum Android SDK target of 26. Update this value in your android/variables.gradle
file:
ext {
minSdkVersion = 26
}
Next, modify the allprojects > repositories
section in your android/build.gradle
file to include the Outsystems repository. Your android/build.gradle
should look like this:
allprojects {
repositories {
google()
mavenCentral()
maven {
url 'https://pkgs.dev.azure.com/OutSystemsRD/9e79bc5b-69b2-4476-9ca5-d67594972a52/_packaging/PublicArtifactRepository/maven/v1'
name 'Azure'
credentials {
username = "optional"
password = ""
}
content {
includeGroup "com.github.outsystems"
}
}
}
}
Step 7: Configure iOS Platform
For iOS, ensure you configure the Privacy - Camera Usage Description in your Info.plist
file to allow your app to access the device’s camera. Add the following entry:
<key>NSCameraUsageDescription</key>
<string>We need access to your camera to scan barcodes.</string>
Step 8: Test Your App
Run your app on a device, as barcode scanning won’t work on a browser. Use the following commands:
ionic capacitor add android
ionic capacitor add ios
ionic capacitor run android
ionic capacitor run ios
Alternatively, build the app and open it in the respective IDE:
ionic capacitor build android
ionic capacitor build ios
Then deploy to a device using Android Studio or Xcode.
Conclusion
By following these steps, you have successfully integrated a barcode scanner into your Ionic app using the Capacitor plugin.
For more posts like this, follow me on LinkedIn or visit my blog Ionic Notes.
Happy coding!