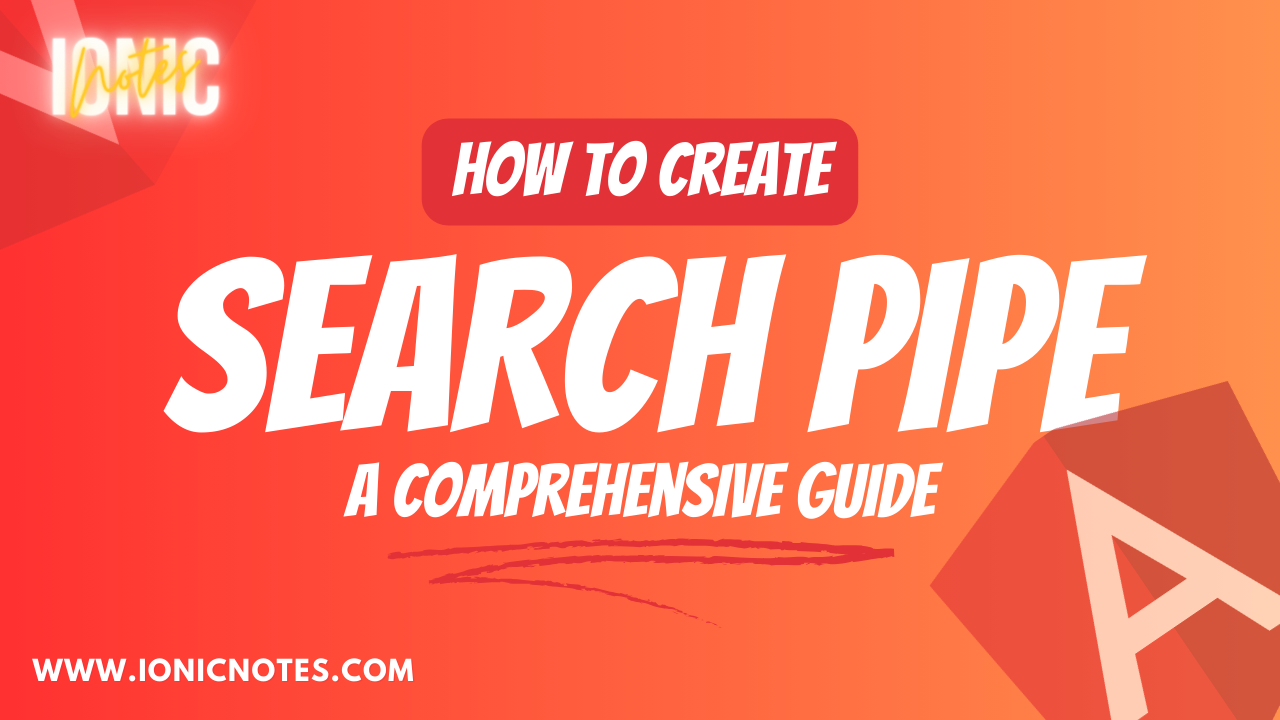
How to create Search Pipe in Angular
- Published on
- 6 mins
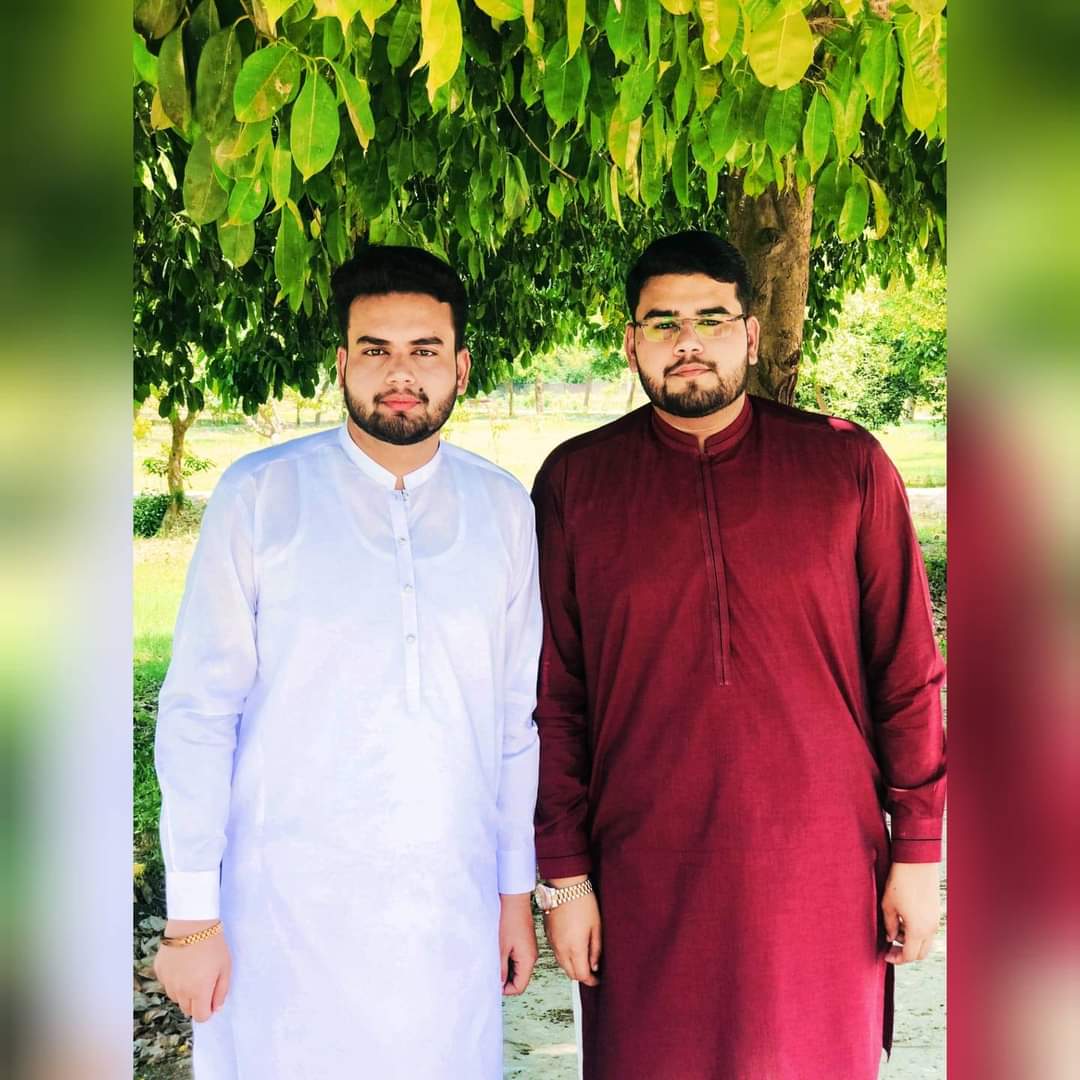
CEO & Founder
Creating an Angular Project and Implementing a Custom Search Pipe
In this tutorial, we will walk through the process of creating an Angular project and implementing a custom search pipe to filter data based on a specific variable.
Step 1: Setting Up the Angular Development Environment
To get started, make sure you have Node.js and npm installed on your system. If not, install them from the official websites.
Next, install the Angular CLI globally by running the following command:
npm install -g @angular/cli
Step 2: Creating a New Angular Project
Create a new Angular project by running the following command:
ng new custom-search-app
Navigate to the project directory:
cd custom-search-app
Step 3: Implementing the Custom Search Pipe
Create a new file called custom-search.pipe.ts
in the app
directory and add the following code:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'customSearch'
})
export class CustomSearchPipe implements PipeTransform {
transform(items: any[], searchText: string, searchKey: string): any[] {
if (!items) {
return [];
}
if (!searchText) {
return items;
}
searchText = searchText.toLowerCase();
return items.filter((item) => {
return item[searchKey].toLowerCase().includes(searchText);
});
}
}
Add the CustomSearchPipe
to your Angular module in app.module.ts
:
import { NgModule } from '@angular/core';
import { CustomSearchPipe } from './custom-search.pipe';
@NgModule({
declarations: [
// other declarations
CustomSearchPipe
]
// other module configurations
})
export class AppModule {}
In your component HTML file, use the custom pipe as follows:
<input type="text" [(ngModel)]="searchText" placeholder="Search" />
<ul>
<li *ngFor="let item of items | customSearch: searchText:searchKey">{{ item.name }}</li>
</ul>
In your component TypeScript file, define the items
array and specify the searchKey
variable:
import { Component } from '@angular/core';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
styleUrls: ['./your-component.component.css']
})
export class YourComponent {
searchText: string = '';
searchKey: string = 'name';
items = [
{ name: 'John' },
{ name: 'Doe' },
{ name: 'Jane' }
// other items
];
}
Step 4: Running the Angular Application
To run the Angular application, use the following command:
ng serve
Visit http://localhost:4200/
in your web browser to see the application in action.
That’s it! You have successfully created an Angular project and implemented a custom search pipe to filter data in the application.
Feel free to adjust the code snippets to fit your specific requirements and customize the Angular application as needed. Must Follow us on Twitter for latest Posts and Articles Alerts