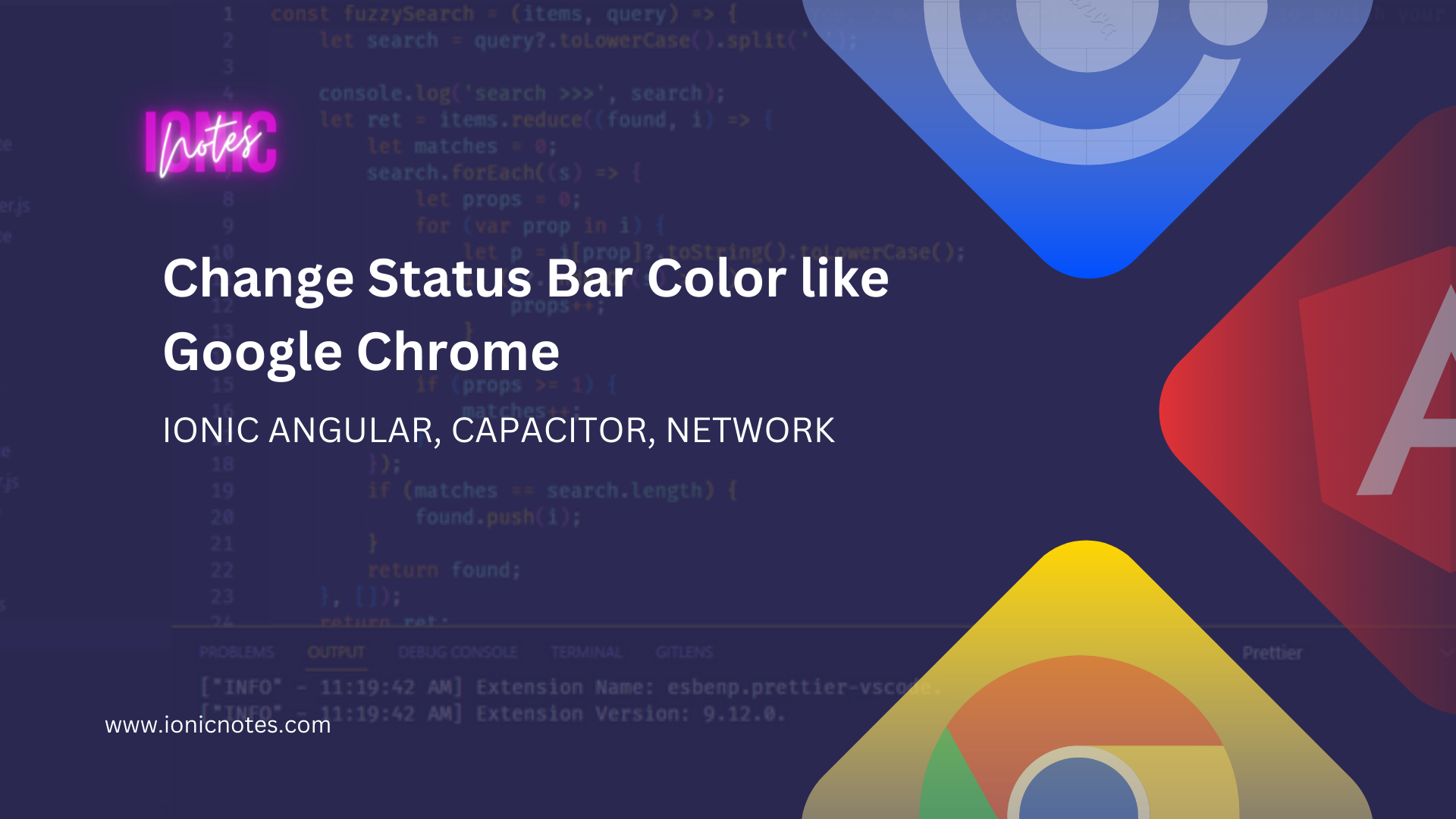
Change Status Bar Color like Google Chrome
- Published on
- 5 mins
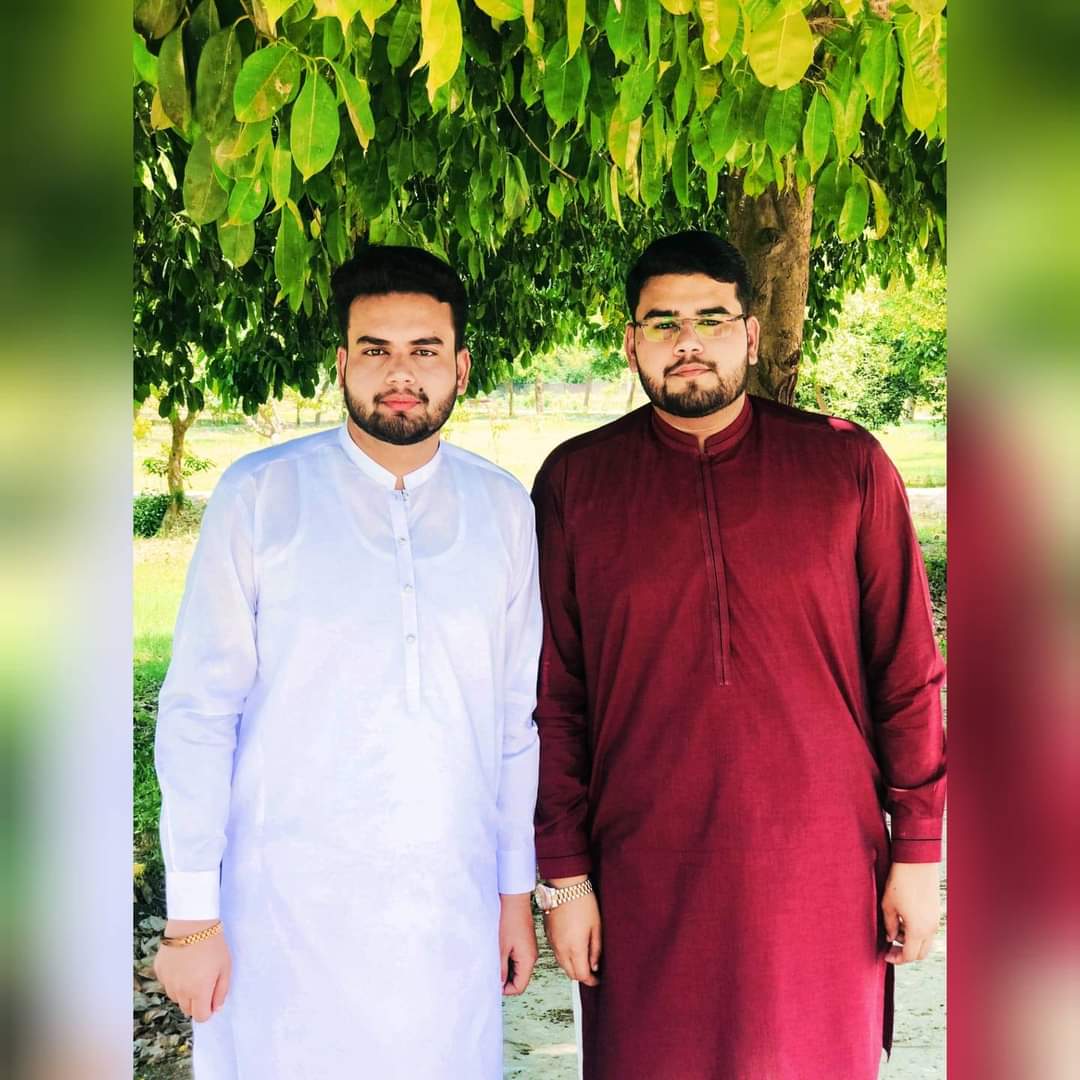
CEO & Founder
Enhancing App User Experience with Capacitor Plugins: Network and Status Bar Integration
In today’s digital age, mobile applications must provide a seamless user experience, even when the network connection is unreliable. By leveraging the power of Capacitor, a powerful cross-platform framework, and incorporating the Capacitor Network and Status Bar plugins, developers can create apps that dynamically respond to network connectivity changes. In this blog post, we will explore how to implement a feature where the status bar color changes when the network disconnects and reconnects, enhancing the overall user experience.
Prerequisites
Before we begin, make sure you have a basic understanding of Angular and Capacitor, as well as a working environment set up for Ionic app development.
Step 1: Set up the Project
Create a new Ionic app using the Angular framework by running the following command:
ionic start my-app blank --type=angular
Navigate into the project directory:
cd my-app
Step 2: Install the Required Plugins
Install the Capacitor Network and Status Bar plugins by running the following commands:
npm install @capacitor/network @capacitor/status-bar
npx cap sync
Step 3: Implement Network Connectivity Detection
Open the app.component.ts
file and import the Capacitor Network plugin:
import { Network } from '@capacitor/network';
import { StatusBar, Style } from '@capacitor/status-bar';
Next, add the following code to the initializeApp()
method:
initializeApp() {
// ...existing code...
// Network connectivity change detection
Network.addListener('networkStatusChange', (status: NetworkStatus) => {
const color = status.connected ? 'blue' : 'red';
StatusBar.setBackgroundColor({ color });
});
// ...existing code...
}
Step 4: Configure the Status Bar Plugin
In the same app.component.ts
file, import the Capacitor Status Bar plugin:
import { Plugins } from '@capacitor/core';
const { StatusBar } = Plugins;
Inside the initializeApp()
method, after the network connectivity change detection code, add the following code to set the initial status bar color:
initializeApp() {
// ...existing code...
// Set initial status bar color
StatusBar.setBackgroundColor({ color: 'blue' });
// ...existing code...
}
Step 5: Build and Run the App
Build the app by running the following command:
ionic build
Once the build process completes, sync the app with your native project:
npx cap copy
Run the app on your desired platform (iOS or Android):
npx cap open ios
or
npx cap open android
Conclusion
By utilizing the Capacitor Network and Status Bar plugins in your Ionic app, you can create a responsive user experience that adapts to network connectivity changes. The integration of these plugins allows you to dynamically change the status bar color to provide immediate visual feedback to the users. With just a few simple steps, you can enhance the overall user experience and ensure your app remains functional even in challenging network conditions.
Remember to consult the official Capacitor documentation for more information on additional features and customization options provided by these plugins. Happy coding!