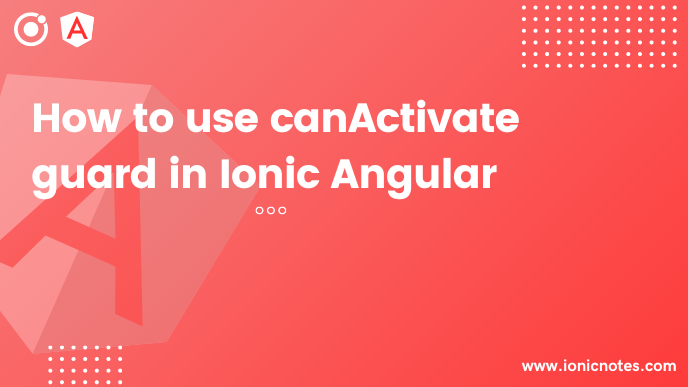
How to use canActivate guard in Ionic Angular
- Published on
- 5 mins
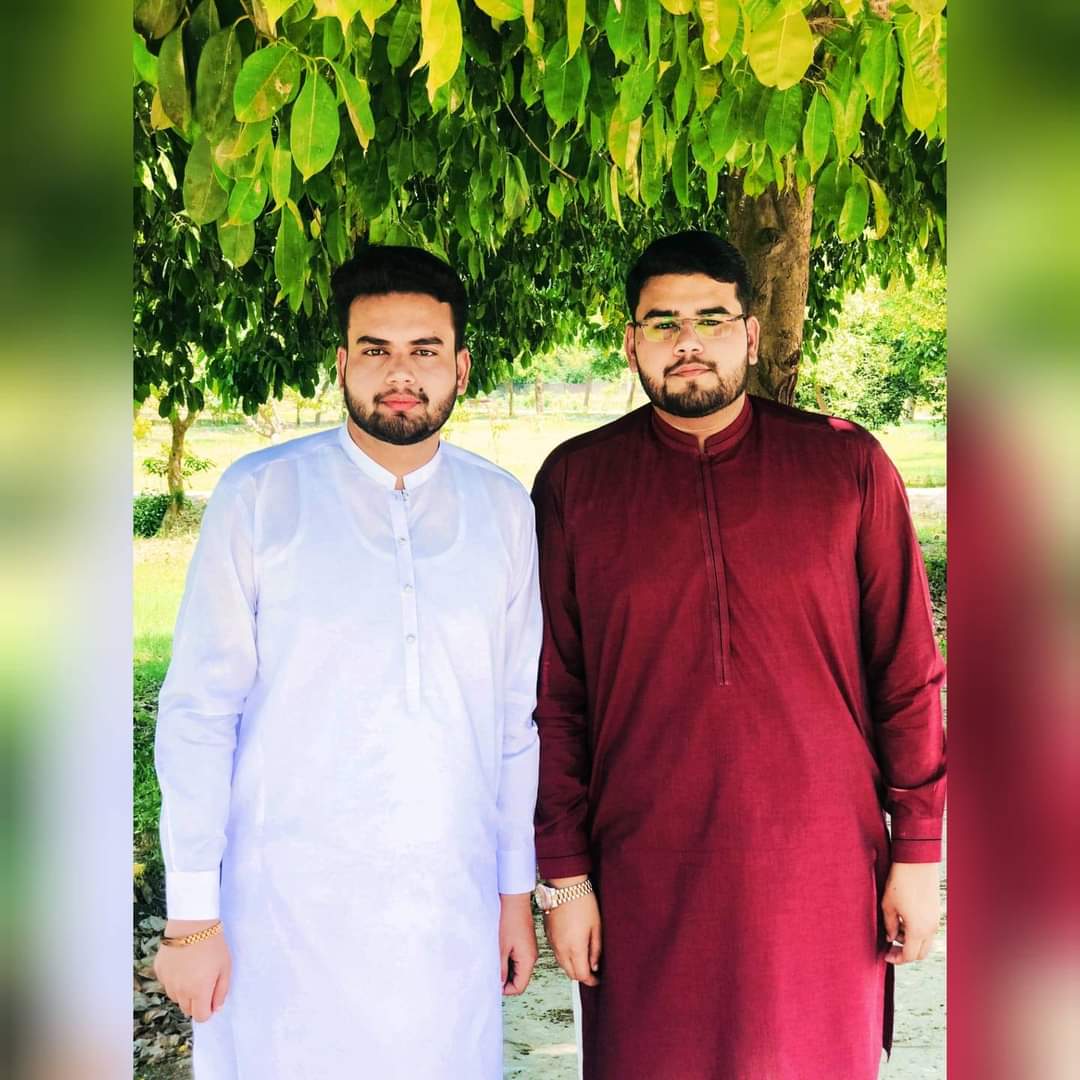
CEO & Founder
What is canActivate Guard?
The canActivate guard is a feature provided by Angular that allows you to control access to specific routes in your application. It allows you to add an authentication check to a route and only allow access if the user is authenticated.
Installing Ionic and Creating a New Project
Before we get started, we need to ensure that we have Ionic and Angular installed. If you haven’t already, please follow these steps:
Install Node.js: Go to the Node.js website and download the latest version. Once it’s downloaded, run the installation file and follow the instructions.
Install Ionic and Cordova: Open your command prompt or terminal and run the following command:
npm install -g ionic cordova
This will install both Ionic and Cordova globally on your system.
- Create a new project: Run the following command to create a new Ionic project:
ionic start myApp tabs
This will create a new Ionic project with a tab-based layout.
Using canActivate Guard in Ionic Angular
Now that we have our project set up, let’s add the canActivate guard to our app.
- Create a new service: Run the following command to create a new service:
ionic g service auth
This will create a new service called auth in your app. This service will be responsible for checking whether the user is authenticated or not.
- Add canActivate guard to the routes: In your app.module.ts file, import the AuthGuard service that we just created:
import { AuthGuard } from './auth.guard';
Now, add the canActivate property to the routes that you want to protect, and set it to the AuthGuard service:
const routes: Routes = [
{
path: 'home',
component: HomePage
},
{
path: 'protected',
component: ProtectedPage,
canActivate: [AuthGuard]
}
];
In this example, we’re protecting the /protected route.
- Implement the canActivate method: In the auth.guard.ts file, we need to implement the canActivate method. This method will be called every time a user tries to access a protected route.
import { Injectable } from '@angular/core';
import {
CanActivate,
ActivatedRouteSnapshot,
RouterStateSnapshot,
UrlTree,
Router
} from '@angular/router';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private router: Router) {}
canActivate(next: ActivatedRouteSnapshot, state: RouterStateSnapshot): boolean {
// Check if the user is authenticated
if (localStorage.getItem('isLoggedIn') === 'true') {
return true;
} else {
this.router.navigate(['/']);
return false;
}
}
}
In this example, we’re checking if the user is authenticated by looking for a localStorage key called isLoggedIn.