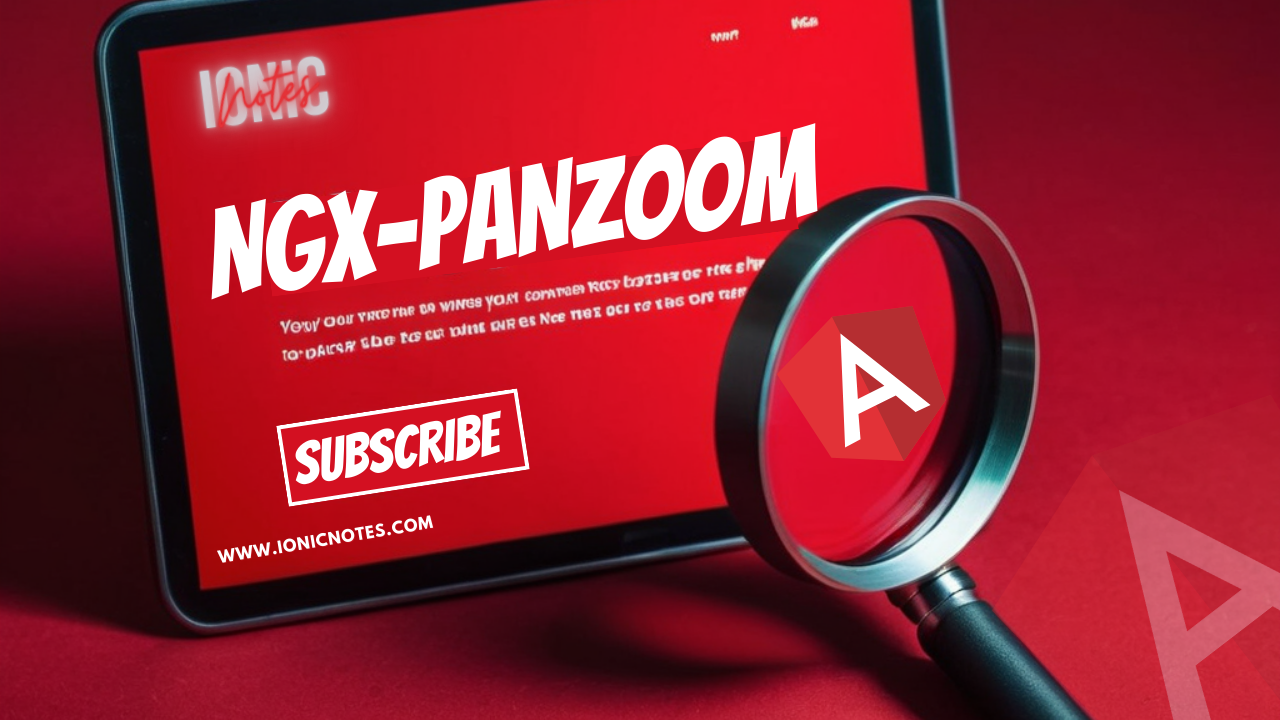
How to use Panzoom in Angular
- Published on
- 7 mins
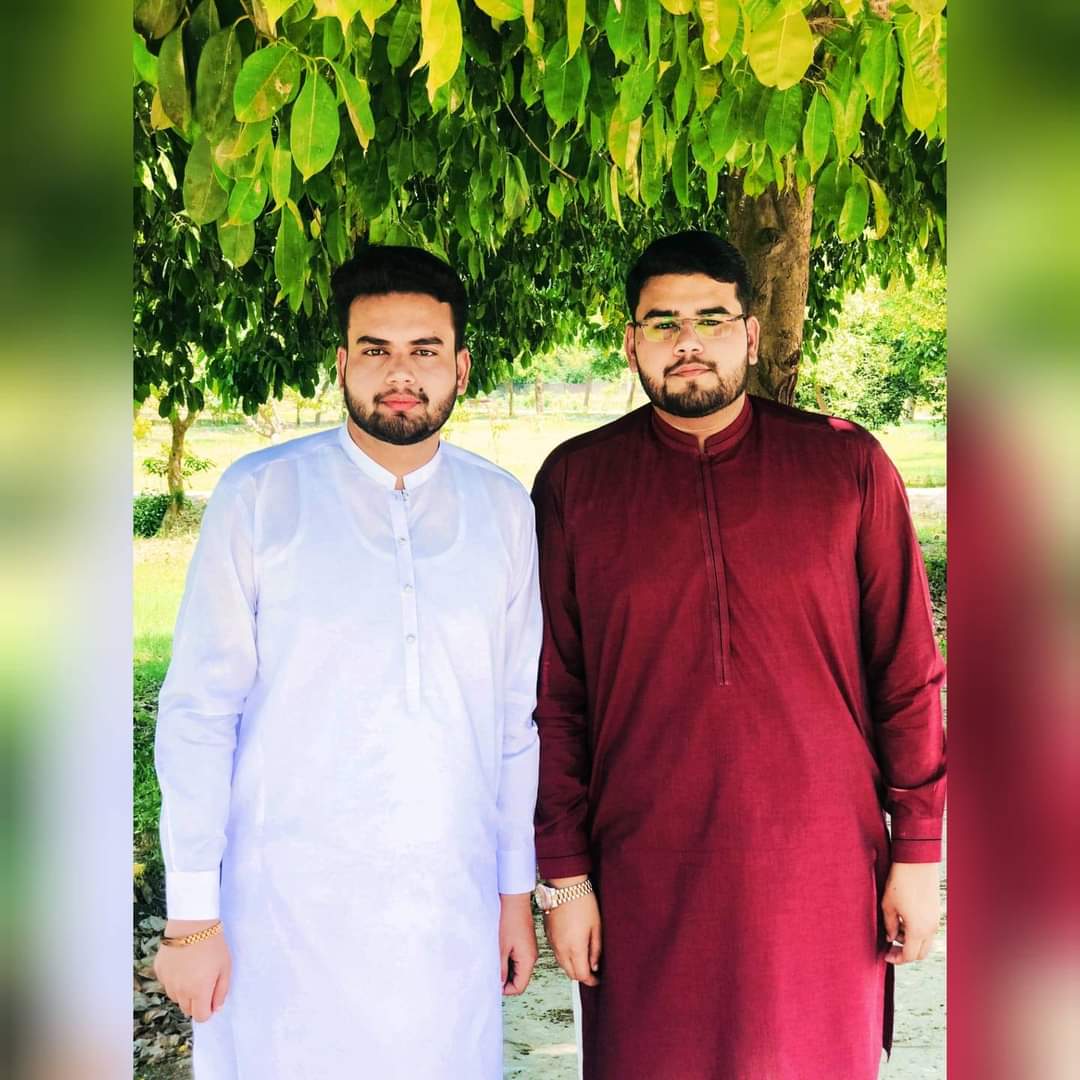
CEO & Founder
Angular Ngx-Panzoom: Enhancing User Experience with Powerful Zooming and Panning
In modern web development, creating an intuitive and user-friendly interface is key to engaging your audience. Angular, a popular JavaScript framework, offers various tools and libraries to simplify complex tasks. One such tool is ngx-panzoom
, which allows you to easily implement zooming and panning functionality within your Angular application.
In this guide, we will explore how to integrate ngx-panzoom
into an Angular project and create a user interface featuring zoom in, zoom out, reset, and fit-to-screen functionalities.
Setting up an Angular Project
To get started, ensure that you have Node.js and npm (Node Package Manager) installed on your system. If not, you can download them from here. Once installed, follow these steps:
- Open your terminal or command prompt.
- Run the following command to install Angular CLI globally if you haven’t already:
npm install -g @angular/cli
- Create a new Angular project by running:
ng new my-ngx-pan-zoom-project
- Navigate to the project directory:
cd my-ngx-pan-zoom-project
- Launch the development server:
ng serve
- Open your browser and navigate to
http://localhost:4200/
to see your new Angular application.
Integrating Ngx-panzoom
To integrate ngx-panzoom
into your Angular project, follow these steps:
Install
ngx-panzoom
by running the following command:npm install ngx-panzoom --save
Import the
NgxPanZoomModule
in your Angular module. Openapp.module.ts
and add the following import statement:import { NgxPanZoomModule } from 'ngx-panzoom'; @NgModule({ imports: [ // Other imports... NgxPanZoomModule ] // Other configurations... }) export class AppModule {}
Creating the User Interface
Now, let’s create a user interface with zoom in, zoom out, reset, and fit-to-screen functionalities in the footer using the panzoomapi
and panzoomconfig
. Here’s a sample implementation:
<!-- app.component.html -->
<div class="main-container">
<div style="position: absolute; top: 100px; bottom: 0; left: 0; right: 0;">
<pan-zoom [config]="panZoomConfig">
<div style="position: relative;">
<img
src="https://image.freepik.com/free-psd/young-girl-with-yellow-sweater-blue-bandana-her-head-extending-hands_1368-19065.jpg"
/>
</div>
</pan-zoom>
</div>
<div class="footer-container">
<button (click)="zoomIn()">Zoom In</button>
<button (click)="zoomOut()">Zoom Out</button>
<button (click)="reset()">Reset</button>
<button (click)="fitToScreen()">Fit to Screen</button>
</div>
</div>
// app.component.ts
import { Component, OnInit, ViewChild } from '@angular/core';
import { NgxPanZoomComponent, PanZoomConfig, PanZoomAPI } from 'ngx-panzoom';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit, OnDestroy {
panZoomConfig: PanZoomConfig = new PanZoomConfig();
private panZoomAPI: PanZoomAPI;
private apiSubscription: Subscription;
ngOnInit(): void {
//Subscribe to PanZoomApi
this.apiSubscription = this.panzoomConfig.api.subscribe(
(api: PanZoomAPI) => (this.panZoomAPI = api)
);
}
zoomIn() {
this.panZoomAPI.zoomIn();
}
zoomOut() {
this.panZoomAPI.zoomOut();
}
reset() {
this.panZoomApi.resetView();
}
fitToScreen() {
this.panZoomApi.centerContent();
}
}
By following these steps, you can easily incorporate ngx-panzoom
into your Angular project and create a user-friendly interface with powerful zooming and panning capabilities.