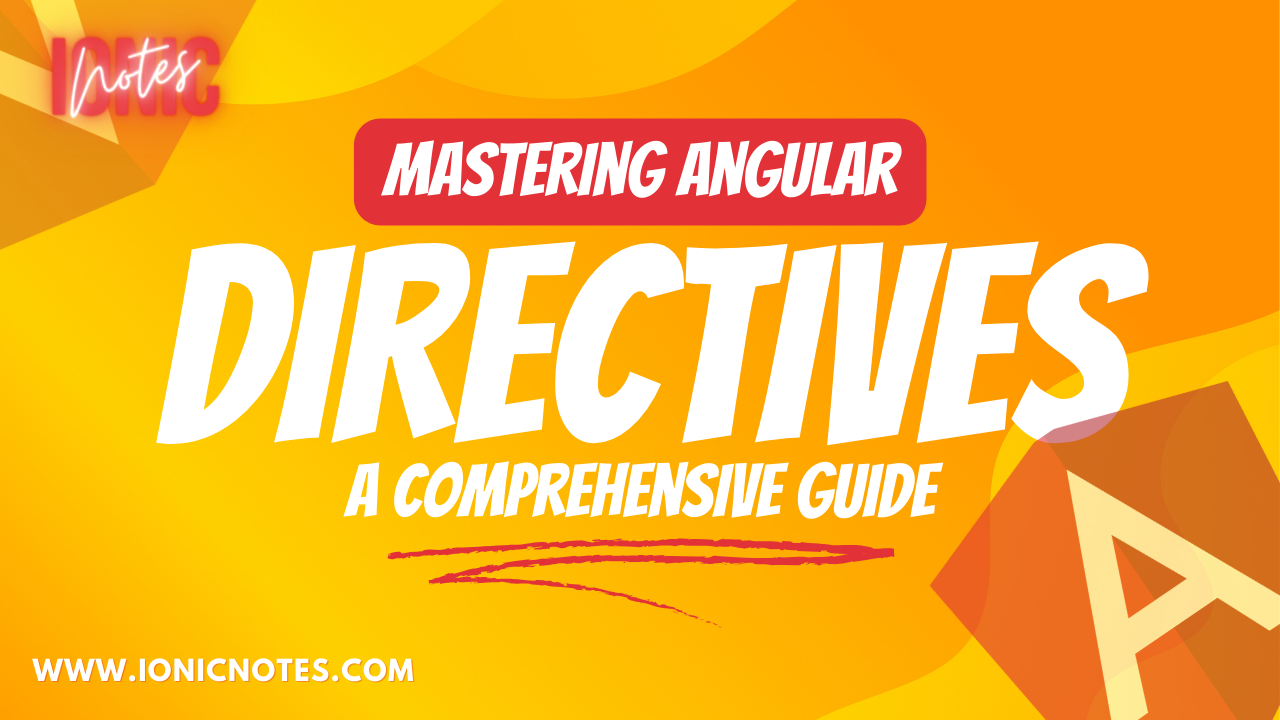
Mastering Angular Directives: A Comprehensive Guide
- Published on
- 5 mins
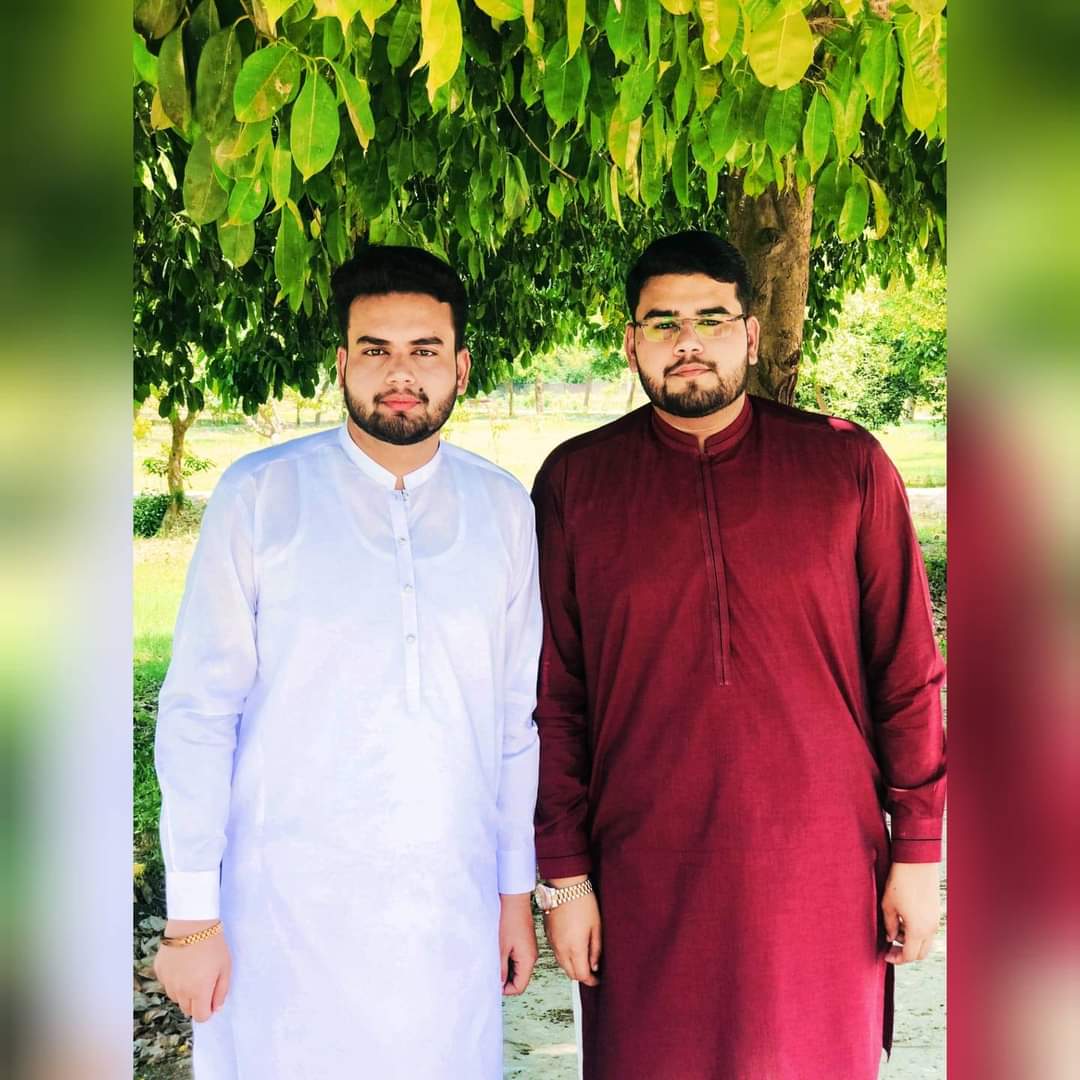
CEO & Founder
Building an Angular App with Custom Directives
In this tutorial, we’ll walk you through the process of creating a new Angular application and then demonstrate how to build and use a custom directive for highlighting elements when hovered.
Prerequisites
Before we get started, make sure you have the following prerequisites installed on your system:
- Node.js and npm (Node Package Manager): You can download them from nodejs.org.
Step 1: Creating a New Angular App
1.1 Install Angular CLI
To create a new Angular application, we’ll use the Angular CLI (Command Line Interface). Open your terminal and install the Angular CLI globally by running the following command:
npm install -g @angular/cli
1.2 Create a New Angular App
Once the Angular CLI is installed, you can create a new Angular app by running the following command:
ng new angular-custom-directive-demo
Follow the prompts to configure your project. You can choose the default options for this demonstration.
1.3 Navigate to the App Directory
Navigate to the newly created app directory:
cd angular-custom-directive-demo
Step 2: Creating a Custom Directive
2.1 Generate the Directive
We’ll now generate a custom directive using the Angular CLI. Run the following command to generate the directive:
ng generate directive highlight
This command will create a new directive file named highlight.directive.ts
and add it to your app.
2.2 Implement the Custom Directive
Now, let’s open the highlight.directive.ts
file and implement the custom directive as follows:
// src/app/highlight.directive.ts
import { Directive, ElementRef, HostListener, Renderer2 } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef, private renderer: Renderer2) {}
@HostListener('mouseenter') onMouseEnter() {
this.renderer.setStyle(this.el.nativeElement, 'background-color', 'yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.renderer.removeStyle(this.el.nativeElement, 'background-color');
}
}
2.3 Using the Custom Directive
Now that we’ve created the custom directive, let’s use it in our app. Open the app.component.html
file and add the following code:
<!-- src/app/app.component.html -->
<div>
<p appHighlight>Hover over me to highlight!</p>
</div>
This code applies the appHighlight
directive to the <p>
element. It will highlight the background color in yellow when you hover over it and remove the highlight when you move the mouse away.
Step 3: Running the Application
3.1 Start the Development Server
To run your Angular app, execute the following command:
ng serve
This command will start the development server, and your app will be available at http://localhost:4200/
.
3.2 View Your App
Open your web browser and navigate to http://localhost:4200/
to view your Angular app. You should see the “Hover over me to highlight!” text. Hover over it to see the custom directive in action.
Conclusion
In this tutorial, we’ve covered the basics of creating a new Angular app, generating a custom directive, and using it to add interactive behavior to elements in your application. Custom directives are a powerful tool for extending Angular’s functionality and enhancing the user experience of your web applications.
Feel free to explore and modify the code to suit your specific needs and create more advanced custom directives for your Angular projects.
Happy coding!